OpenGL Programming for Windows MFC
4.Texture
4.1 Texture として使用する BMP ファイルを用意
tiger.bmp
cow.bmp
Prepares a BMP file for use as Texture.
4.2 XXXView.h に以下のメンバー変数追加
CDC* m_pDC ; // Device Context
HGLRC m_GLRC ; // OpenGL Rendering Context
GLfloat m_rAngle ; // Rotation angle
AUX_RGBImageRec* m_pRGBImage ; // RGB image area pointer
This member variable addition to XXXView.h.
4.3 OnCreate を以下のように変更
int CXXXView::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CView::OnCreate(lpCreateStruct) == -1)
return -1;
m_pDC = new CClientDC(this) ; // Get device context
SetDCPixelFormat(m_pDC->m_hDC) ; // Set OpenGL pixel format
m_GLRC = wglCreateContext (m_pDC->m_hDC); // Create rendering context
wglMakeCurrent (m_pDC->m_hDC, m_GLRC); // Current context set
InitGL() ; // Initalize OpenGL
// DIB Image load
m_pRGBImage = auxDIBImageLoad("tiger.bmp"); // Tiger BMP
//m_pRGBImage = auxDIBImageLoad("cow.bmp"); // Cow BMP
// Texture setup
TexGL(m_pRGBImage->sizeX, m_pRGBImage->sizeY, m_pRGBImage->data) ;
return 0;
}
OnCreate is changed as mentioned above.
4.4 OnDestroy を以下のように変更
void CXXXView::OnDestroy()
{
CView::OnDestroy();
free( m_pRGBImage->data) ; // free DIB image area
free( m_pRGBImage );
wglMakeCurrent (NULL, NULL); // free current context
wglDeleteContext (m_GLRC); // Delete rendering context
delete m_pDC ; // Release device context
}
OnDestroy is changed as mentioned above.
4.5 TexGL
GLint TexGL(GLsizei width, GLsizei height, const GLvoid *pixels)
{
glNewList(2, GL_COMPILE) ;
glPixelStorei(GL_UNPACK_ALIGNMENT, 1) ;
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S , GL_REPEAT) ;
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T , GL_REPEAT) ;
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR) ;
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR) ;
glTexEnvi(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE) ;
glTexImage2D(GL_TEXTURE_2D, 0, 3, width, height,
0, GL_RGB, GL_UNSIGNED_BYTE,pixels) ;
glEndList() ;
return(0) ;
}
4.6 DrawGL を以下のように変更
GLint DrawGL(GLfloat rAngle)
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) ;
glMatrixMode(GL_MODELVIEW) ;
glLoadIdentity() ;
glTranslated(0.0, 0.0, -3.0) ;
glRotatef(rAngle, 0.0f, 1.0f, 0.0f) ;
glEnable(GL_TEXTURE_2D ) ;
glCallList(2) ; // Texture
glEnable(GL_NORMALIZE) ;
glEnable(GL_AUTO_NORMAL) ;
auxSolidTeapot(1.0) ;
glDisable(GL_AUTO_NORMAL) ;
glDisable(GL_NORMALIZE) ;
glDisable(GL_TEXTURE_2D ) ;
return(0) ;
}
OnDraw is changed as mentioned above.
4.7 Build and Execute
ビルド・実行すると、Texture Teapotオブジェクトが回転する。
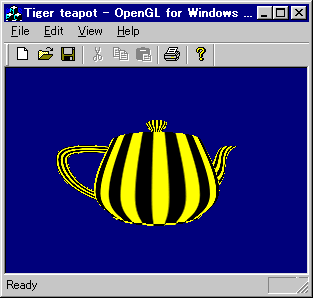
A Texture Teapot object rotates.
prev next